Installing and Using FFmpeg in Python
Introduction
FFmpeg is a powerful multimedia framework that can decode, encode, transcode, mux, demux, stream, filter, and play just about anything that humans and machines have created. It supports the most obscure ancient formats up to the cutting edge. This versatility makes it an invaluable tool for developers dealing with multimedia data.
In this blog, we will cover how to install FFmpeg and use it in Python. We will use the ffmpeg-python
library, which is a Python binding for FFmpeg, making it easier to work with FFmpeg commands in Python.
Installing FFmpeg
Before we can use FFmpeg in Python, we need to install the FFmpeg binary on our system.
Installing FFmpeg on Windows
- Download the FFmpeg executable from the official FFmpeg website.
- Extract the downloaded zip file.
- Add the
bin
directory of the extracted files to your system’s PATH environment variable.
Installing FFmpeg on macOS
You can use Homebrew to install FFmpeg:
Installing FFmpeg on Linux
On Ubuntu or Debian-based systems, you can install FFmpeg using:
sudo apt update
sudo apt install ffmpeg

On Fedora, you can use:

Installing ffmpeg-python
With FFmpeg installed, we can now install the ffmpeg-python
library. This library provides a more Pythonic interface to FFmpeg.
Install it using pip:
pip install ffmpeg-python

Using FFmpeg in Python
Here’s a basic example of how to use FFmpeg in Python to convert a video file from one format to another.
Converting a Video File
output_file = 'output.avi'
ffmpeg.input(input_file).output(output_file).run()
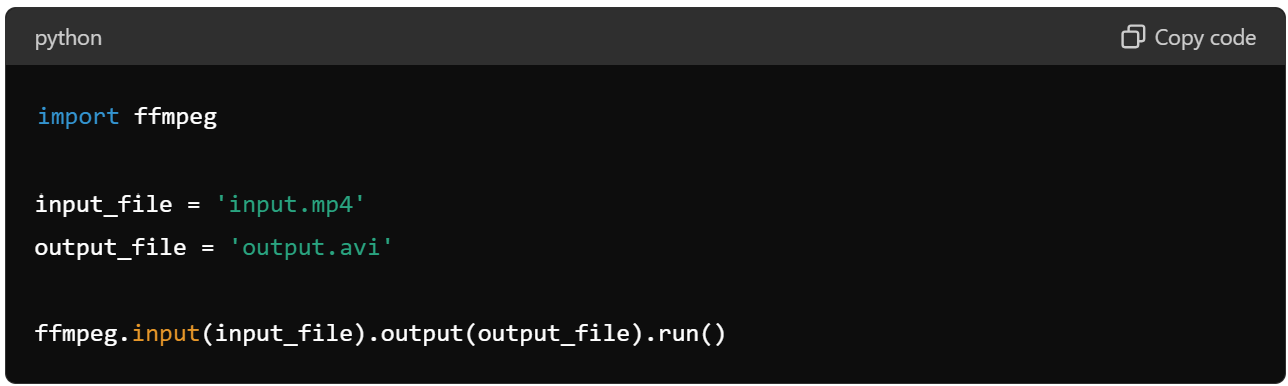
Extracting Audio from a Video File
You might want to extract the audio from a video file. Here’s how you can do it:
output_file = 'output.mp3'
ffmpeg.input(input_file).output(output_file).run()
Resizing a Video
You can also resize a video using FFmpeg:
output_file = 'output_resized.mp4'
ffmpeg.input(input_file).output(output_file, vf='scale=1280:720').run()
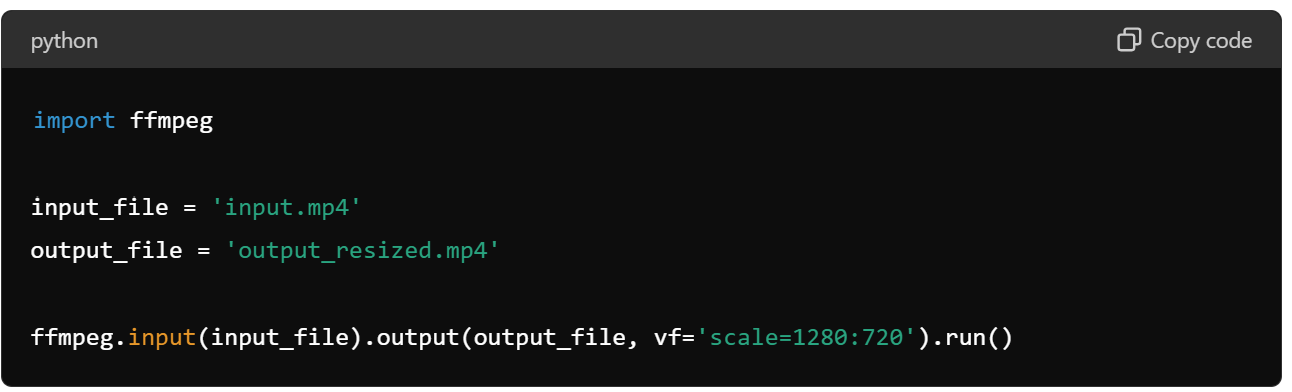
Adding a Watermark to a Video
To add a watermark to a video, you can use the following code:
watermark = 'watermark.png'
output_file = 'output_with_watermark.mp4'
ffmpeg.input(input_file).output(output_file, vf='movie=watermark.png [watermark]; [in][watermark] overlay=W-w-10:H-h-10 [out]').run()
Combining Multiple Commands
FFmpeg is very powerful and allows you to chain multiple commands together. Here’s an example where we convert a video, resize it, and extract the audio all in one go:
video_output_file = 'output_resized.avi'
audio_output_file = 'output_audio.mp3'
ffmpeg.input(input_file).output(video_output_file, vf='scale=1280:720').run()
ffmpeg.input(input_file).output(audio_output_file).run()
Error Handling
FFmpeg commands might fail for various reasons (e.g., invalid input files, missing codecs). The ffmpeg-python
library provides a way to catch and handle these errors.
output_file = 'output.avi'
try:
ffmpeg.input(input_file).output(output_file).run()
except ffmpeg.Error as e:
print(f"An error occurred: {e.stderr.decode()}")
Conclusion
FFmpeg is a versatile tool that can handle a wide range of multimedia tasks. By using the ffmpeg-python
library, we can leverage the power of FFmpeg in a more Pythonic way. This blog covered basic usage examples, but FFmpeg’s capabilities are vast. I encourage you to explore the FFmpeg documentation and the ffmpeg-python GitHub page for more advanced usage and examples.
Happy coding!